As part of our commitment to our customers, we provide a free lifetime warranty on defective parts and labor. Though each warranty is handled on a case by case basis, we stand by the notion of doing business reasonable and fairly. Why so many customers choose Glass Act Windshield Services is because we are very confident in our workmanship once completed. If you need further information or the need to discuss the warranty, please email at Jeff@glassactaz.com or call 480-208-9028.
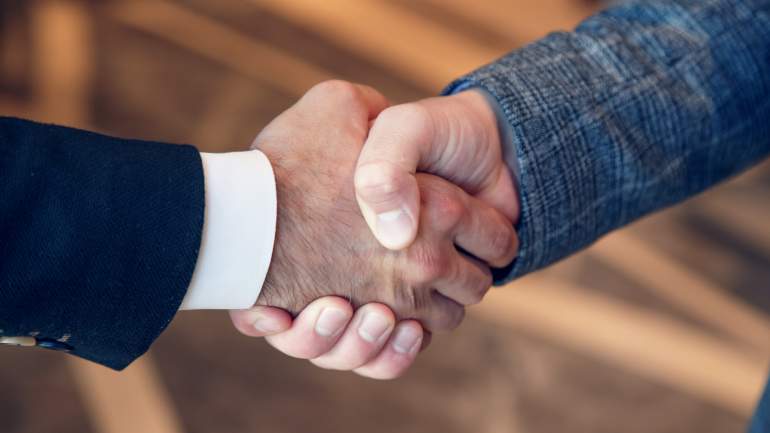